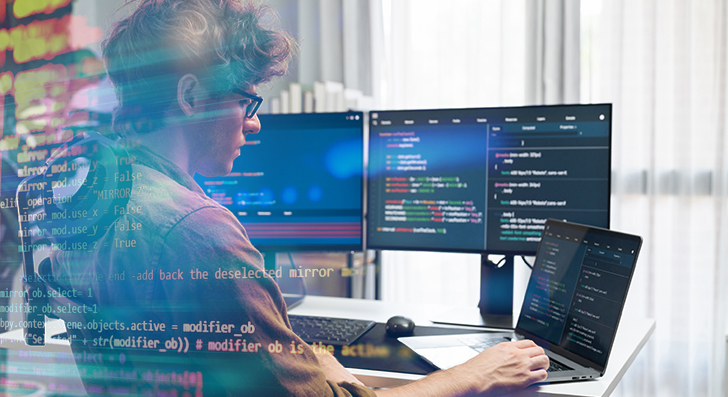
Scalability indicates your application can manage development—more people, much more data, plus more website traffic—devoid of breaking. Like a developer, building with scalability in your mind saves time and strain afterwards. Right here’s a transparent and functional manual to help you start out by Gustavo Woltmann.
Style for Scalability from the beginning
Scalability is not a little something you bolt on afterwards—it should be aspect of one's approach from the beginning. Lots of programs are unsuccessful after they develop rapid since the first style can’t cope with the extra load. Being a developer, you need to Feel early regarding how your technique will behave stressed.
Commence by coming up with your architecture to become versatile. Stay clear of monolithic codebases in which anything is tightly connected. In its place, use modular style or microservices. These designs crack your application into smaller sized, impartial sections. Every module or support can scale By itself without having impacting The complete system.
Also, take into consideration your database from working day a person. Will it require to deal with 1,000,000 users or perhaps a hundred? Select the suitable style—relational or NoSQL—based on how your information will expand. Prepare for sharding, indexing, and backups early, Even when you don’t require them but.
Yet another significant point is to avoid hardcoding assumptions. Don’t create code that only operates beneath recent ailments. Think of what would come about If the person foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use design patterns that assist scaling, like information queues or event-driven programs. These enable your application tackle extra requests without the need of obtaining overloaded.
After you Create with scalability in mind, you're not just getting ready for success—you're reducing upcoming complications. A very well-prepared system is less complicated to take care of, adapt, and increase. It’s far better to prepare early than to rebuild later.
Use the ideal Databases
Selecting the right database is really a key Element of making scalable programs. Not all databases are constructed the same, and utilizing the Mistaken one can gradual you down or simply lead to failures as your app grows.
Get started by comprehension your info. Can it be hugely structured, like rows inside a desk? If Indeed, a relational database like PostgreSQL or MySQL is a good healthy. These are generally powerful with interactions, transactions, and consistency. In addition they assist scaling techniques like examine replicas, indexing, and partitioning to deal with extra targeted visitors and info.
If your knowledge is more versatile—like person activity logs, product or service catalogs, or documents—look at a NoSQL choice like MongoDB, Cassandra, or DynamoDB. NoSQL databases are better at dealing with significant volumes of unstructured or semi-structured info and will scale horizontally much more quickly.
Also, consider your read through and generate patterns. Are you presently executing lots of reads with less writes? Use caching and browse replicas. Are you dealing with a significant write load? Explore databases which can deal with substantial create throughput, as well as party-based info storage programs like Apache Kafka (for non permanent information streams).
It’s also wise to Consider in advance. You might not need State-of-the-art scaling options now, but choosing a database that supports them implies you gained’t need to have to change afterwards.
Use indexing to hurry up queries. Stay clear of unnecessary joins. Normalize or denormalize your information determined by your obtain styles. And always keep track of database overall performance as you expand.
In a nutshell, the best database relies on your application’s composition, pace demands, And just how you assume it to increase. Just take time to choose properly—it’ll conserve lots of difficulty later on.
Optimize Code and Queries
Quick code is key to scalability. As your application grows, just about every smaller delay adds up. Poorly penned code or unoptimized queries can decelerate functionality and overload your procedure. That’s why it’s imperative that you Make productive logic from the start.
Start by crafting cleanse, straightforward code. Stay away from repeating logic and take away nearly anything unneeded. Don’t choose the most sophisticated solution if a straightforward just one will work. Maintain your capabilities limited, targeted, and straightforward to test. Use profiling tools to search out bottlenecks—sites the place your code requires much too prolonged to run or works by using a lot of memory.
Next, check out your database queries. These generally slow points down in excess of the code itself. Ensure that Each and every question only asks for the data you truly require. Prevent Choose *, which fetches all the things, and as an alternative find certain fields. Use indexes to hurry up lookups. And keep away from accomplishing too many joins, In particular throughout huge tables.
For those who discover the exact same information currently being asked for again and again, use caching. Keep the outcomes briefly applying resources like Redis or Memcached therefore you don’t have to repeat pricey functions.
Also, batch your databases functions whenever you can. As an alternative to updating a row one after the other, update them in teams. This cuts down on overhead and tends to make your application much more successful.
Make sure to test with huge datasets. Code and queries that get the job done great with 100 information could crash every time they have to handle 1 million.
In brief, scalable applications are speedy applications. Keep the code limited, your queries lean, and use caching when needed. These actions aid your application remain easy and responsive, whilst the load will increase.
Leverage Load Balancing and Caching
As your app grows, it's to take care of much more consumers and a lot more website traffic. If anything goes by a person server, it will eventually immediately turn into a bottleneck. That’s wherever load balancing and caching can be found in. Both of these equipment aid keep the application rapidly, steady, and scalable.
Load balancing spreads incoming site visitors across multiple servers. Instead of a person server accomplishing many of the get the job done, the load balancer routes people to diverse servers depending on availability. This means no solitary server will get overloaded. If 1 server goes down, the load balancer can send visitors to the Other folks. Equipment like Nginx, HAProxy, or cloud-based methods from AWS and Google Cloud make this very easy to create.
Caching is about storing information quickly so it could be reused rapidly. When users request the exact same data once more—like an item website page or even a profile—you don’t need to fetch it within the databases each and every time. You can provide it in the cache.
There's two widespread kinds of caching:
1. Server-aspect caching (like Redis or Memcached) retailers details in memory for rapidly access.
two. Client-aspect caching (like browser caching or CDN caching) shops static data files close to the person.
Caching decreases databases load, enhances velocity, and would make your app additional effective.
Use caching for things which don’t alter generally. And usually ensure that your cache is updated when facts does change.
In a nutshell, load balancing and caching are simple but strong applications. With each other, they assist your app tackle much more buyers, remain rapid, and recover from difficulties. If you intend to mature, you'll need equally.
Use Cloud and Container Tools
To construct scalable apps, you would like tools that let your application develop simply. That’s wherever cloud platforms and containers come in. They give you overall flexibility, cut down setup time, and make scaling Significantly smoother.
Cloud platforms like Amazon World wide web Products and services (AWS), Google Cloud System (GCP), and Microsoft Azure let you rent servers and providers as you may need them. You don’t really have to buy hardware or guess foreseeable future potential. When traffic increases, you are able to incorporate far more means with just a few clicks or automatically utilizing automobile-scaling. When targeted traffic drops, you may scale down to economize.
These platforms also present expert services like managed databases, storage, load balancing, and stability resources. You'll be able to target making your app as an alternative to controlling infrastructure.
Containers are One more essential Instrument. A container packages your app and everything it really should operate—code, libraries, options—into a single unit. This can make it uncomplicated to maneuver your application among environments, from your notebook on the cloud, with no surprises. Docker is the most popular tool for this.
Once your app uses various containers, instruments like Kubernetes enable you to manage them. Kubernetes handles deployment, scaling, and Restoration. If one particular component within your application crashes, it restarts it mechanically.
Containers also allow it to be easy to individual elements of your application into providers. You are able to update or scale sections independently, which can be perfect for functionality and reliability.
Briefly, making use of cloud and container applications implies you can scale rapidly, deploy effortlessly, and Get well speedily when problems come about. If you would like your application to grow with no restrictions, commence applying these resources early. They help you save time, decrease possibility, and help you remain centered on building, not repairing.
Watch Every thing
In case you don’t observe your software, you received’t know when things go Mistaken. Checking helps you see how your app is undertaking, location problems early, and make far better selections as your application grows. It’s a key Portion of constructing scalable units.
Begin by tracking simple metrics like CPU utilization, memory, disk House, and reaction time. These tell you how your servers and companies are accomplishing. Tools like Prometheus, Grafana, Datadog, or New Relic will help you gather and visualize this knowledge.
Don’t just watch your servers—observe your application too. Keep an eye on how long it will take for customers to load webpages, how often mistakes take place, and the place they arise. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring within your code.
Put in place alerts for vital complications. For example, if your more info reaction time goes higher than a Restrict or maybe a assistance goes down, it is best to get notified promptly. This will help you correct concerns quickly, frequently prior to users even see.
Checking can be beneficial whenever you make modifications. If you deploy a completely new element and find out a spike in problems or slowdowns, you'll be able to roll it back right before it brings about actual damage.
As your application grows, targeted traffic and information maximize. Devoid of monitoring, you’ll pass up signs of difficulty right until it’s way too late. But with the proper applications in position, you continue to be in control.
In short, checking assists you keep the app reliable and scalable. It’s not almost spotting failures—it’s about knowledge your program and ensuring that it works perfectly, even under pressure.
Remaining Ideas
Scalability isn’t only for large providers. Even tiny applications require a robust foundation. By planning carefully, optimizing correctly, and utilizing the correct instruments, you are able to Make apps that expand effortlessly with out breaking stressed. Commence smaller, Believe massive, and Establish wise.